Features
Premium video tutorials
Award-winning instructors
Personalized learning
Get certified
Learn at your own pace
Mobile (learn on-the-go)
Unlimited tests and quizzes
Regularly updated content
Overview
C# is a powerful cross-platform, object-oriented programming language used by many programmers to create apps for mobile and desktop.
Designed for beginners, this intro to C# course teaches you the basics of C# programming, including variables, data types, and math operations. You will build on this foundation with practical, hands-on exercises and examples every step of the way. You will be able to apply your skills to create programs of your own - like a fun Madlib game - that you can add to your portfolio. By the end of the course, you will have gained the fundamental knowledge you need to start creating more projects with C#.
In this intro to C# course, you will learn how to:
- Explore fundamental concepts including variables, data types, strings, and math operations
- Collect user input
- Work with arrays, methods, logic, and loops
- Ensure proper error and exception handling
- Get an introduction to classes
- Build your own basic program and more!
Once enrolled, our friendly support team and tutors are here to help with any course-related inquiries.
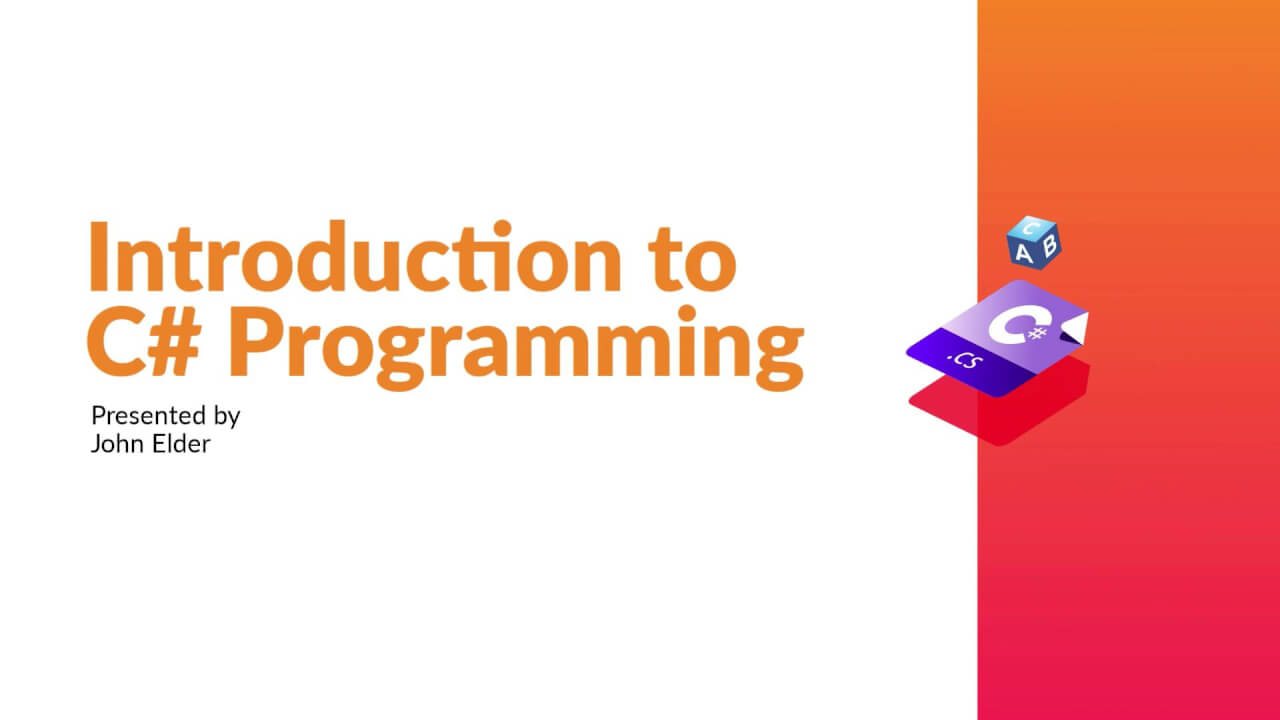
- HD
- 720p
- 540p
- 360p
- 0.50x
- 0.75x
- 1.00x
- 1.25x
- 1.50x
- 1.75x
- 2.00x
Summary
Instructor
Syllabus
Setup And Installation Free Lesson
1
2
Visual Studio Community Installation And Setup
Walking through the process of installing and setting up Visual Studio Community
3
Visual Studio Walkthrough
A quick walkthrough of Visual Studio and some of its basic menus and features
4
First Program: Hello World
We see what happens when we run our first program, "Hello World"
C# Fundamental Programming Concepts Free Lesson
1
2
Data Types: Strings, Char, Int, float, double, decimal, boolean
We introduce many of the Data Types you can use: Strings, Char, Int, float, double, decimal, and boolean.
3
Datetime
We introduce the Datetime data type and explain how to set it to a specific value.
4
DateTime Functions
Let's explore the various functions we can use to display and use the DateTime data type.
5
Null / Nullables
We explain the concept of Null, discuss which fields can have a null value, and introduce Nullables.
6
String Methods
We examine various String Methods to modify string variables, including changing the case and replacing parts of the string.
7
String Indexing
We explain the concept of String Indexing to manage and modify elements within a string.
8
String Concatenation and Interpolation
We learn the difference between Concatenation and Interpolation, and when one might be easier to use than the other.
9
Math (Addition, Subtraction, Multiplication, Division)
Let's learn how to perform basic math functions: Addition, Subtraction, Multiplication, Division.
10
Math (Exponents and Modulus)
In this lesson, we examine how Exponents work and what the Modulus is.
11
Math Order Of Operations
We review PEMDAS and how the Order of Operations works within C#.
12
Math Floats Vs. Ints
In this lesson, we discuss the differences between integers, floats, decimals, and doubles, and how decimal places may be affected based on the data type you choose.
13
Math Incrementation ++ and --
We learn how to increment and decrement variables using ++ and -- commands.
14
Math Methods
In this lesson, we learn about Math Methods such as Floor, Ceiling, Round, and Truncate.
C# Intermediate Programming Concepts Free Lesson
1
Converting Integers To Strings
In this lesson, we discuss how to convert Integers to Strings using the toString method.
2
Type Conversion/Casting
We examine the process for Type Conversion and Casting.
3
User Input / Output
In this lesson, we learn how to capture User Input, assign it to a variable, and Output it to the screen.
4
Build a Madlib Program!
Let's practice our skills with user input to build a Madlib Program!
5
Creating and Accessing Arrays
In this lesson, we learn how to create and access Arrays.
6
Updating Arrays
We explore how to update an existing Array by changing one of its values.
7
Appending Arrays
Appending Arrays in C# is a bit different than in other programming languages.
8
Two Dimensional Arrays
In this lesson, we examine how to create and use Two Dimensional Arrays.
9
Methods
In this lesson, we introduce Methods and how to use them in your program.
10
Passing Parameters To Methods
Once you've created a method, you may want to pass Parameters to those Methods to perform specific operations.
11
Return Methods
If you'd like to use Methods and return data to your program, we explore how that's done.
12
Logic: Comparison Operators (>, >=, <, <=, !=, ==)
In this lesson, we learn about Logic and basic Comparison Operators: >, >=, <, <=, !=, ==.
13
Logic: If/Else Statements
We introduce If/Else Statements and look at how they run inside a program.
14
Logic: If/Else And Operators
In this lesson, we learn how to use "And" operators within an If/Else statement.
15
Logic: If/Else Or Operators
We learn how to use "or" operators within If/Else statements.
16
Logic: If/Else If
In this lesson, we learn how to use "else if" within an If/Else statement.
17
Switch Statement
We look at how to use a Switch Statement within our program.
18
Loops: While Loops
In this lesson, we introduce Loops and explain how While and Do While work.
19
Loops: For Loops
We learn how to create and use For Loops within our program.
20
Loops: Break and Continue
In this lesson, we explain how to Break and Continue loops.
C# Error Handling
1
Error and Exception Handling
We discuss how to deal with Errors within your code and how Exception Handling can prevent your program from crashing.
2
Catching Specific Exceptions
By anticipating specific kinds of Exceptions, you can program your code to treat them in unique ways without crashing.
3
Finally Exception Handling
The Finally part of Exception Handling will ensure specific code runs after try / catch blocks.
4
Exception Lists
In this lesson, we identify a helpful Exception List that can help educate you on potential issues that could impact your code.
C# Object Oriented Classes
1
Intro To Classes (Part 1)
Let's begin a discussion of object-oriented programming so we can create our own data types.
2
Intro To Classes (Part 2)
We learn how to create our own class and define the attributes within.
3
Intro To Classes: Constructors
Constructors provide a simple way to create new objects within our code.
4
Intro To Classes: Object Methods
In this lesson, we learn how to create Object Methods that operate inside your own classes.