Introduction to PHP
Introduction to PHP
Skills you’ll gain
PHP is one of the most popular server-side languages, and plays a huge role in web development. This introductory course can help you learn to use PHP to produce your own dynamic, interactive websites.
Designed for beginners, no prior experience or special skills are required to take this course. You will learn the basics of PHP for web development through hands-on application and examples every step of the way. By the end of the course, you will have built a fully functional math flashcard app and templated out a website to demonstrate your practical knowledge.
Highlights:
- 39 practical tutorials.
- Understand the fundamentals of how PHP works and the client/server relationship.
- How to set up your development environment.
- Back up your code and set up version control with Github.
- Learn the different data types for PHP, including string, integer, float, and boolean.
- How to use arithmetic, assignment, comparison, increment, logic and string operators.
- Use if/else/elseif statements to test against conditions.
- Understand basic arrays and how to sort arrays the quick and easy way.
- How to process HTML Forms with GET vs POST.
- Create and issue cookies to your website visitors.
- Pass information between pages with Sessions.
- Put together everything you've learned by building a math flashcard app and templating out a website.
Once enrolled, our friendly support team and tutors are here to help with any course related inquiries.
Syllabus
Download syllabus-
1
Variables Understanding Variables in PHP. 3m
-
2
Data Types Learn the different data types for PHP, including String, Integer, Float, and Boolean. 3m
-
3
Arithmetic Operators Learn how to do math in PHP. 3m
-
4
Assignment Operators How to assign things in PHP. 3m
-
5
Comparison Operators Comparing two or more things is easy with comparison operators. 3m
-
6
Increment Operators Sometimes you can't bother with math - use increment operators instead. 2m
-
7
Logic Operators Logic sits at the core of all computer programming. Understanding logic operators in PHP. 4m
-
8
String Operators Understanding concatenation and concatenating assignment operators. 3m
-
9
Conditional Statements Learn how to use if/else/elseif statements to test against conditions. 4m
-
10
Switch How to switch between different cases. 3m
-
11
While Loops How to use the While loop. 3m
-
12
Let's Build FizzBuzz! FizzBuzz! is a popular interview quiz question. Let's build it! 4m
-
13
Arrays Understanding basic Arrays. 4m
-
14
Sorting Arrays Learn how to Sort Arrays the quick and easy way. 4m
-
15
Functions How to create and use functions in PHP. 3m
-
16
Random Numbers and Dates Automatically generate random numbers and dates. 4m
-
17
String Manipulation Fun with string manipulation! 4m
-
18
Include Function How to include things from other files in your PHP program. 3m
-
19
Require Function Similar to "Include", but used for a slightly different reason. 3m
-
20
Form Handling - GET vs POST How to process HTML Forms with PHP. 4m
-
21
Superglobals What are Superglobals, and how do you use them? 5m
-
22
Cookies Creating and issuing Cookies to your website visitors. 4m
-
23
Sessions Passing information between pages with Sessions. 3m
-
24
For Loops How to use For Loops. 3m
-
1
Flashcard App Introduction and Setup Let's build a cool math flashcard app! 4m
-
2
Creating the Index Page and Form Let's create the main skeleton of our Flashcard App. 3m
-
3
Making the Form Work Let's build out the form to make it work. 3m
-
4
Right and Wrong Logic Let's write some code to determine if the answer is right or wrong. 3m
-
5
Build out the Other Math Pages Addition works, now let's build pages for Subtraction, Multiplication, and Division. 4m
-
1
Understanding the Templating Concept Understanding how Wordpress uses Templates and how we can do the same thing. 3m
-
2
Templating Links and Headers Let's build out a template for our Header and links. 3m
-
3
Templating with Require How to use Require to template things. 4m
-
4
Finishing Up Let's do a few odds and ends to finish up. 3m
Certificate
Certificate of Completion
Awarded upon successful completion of the course.
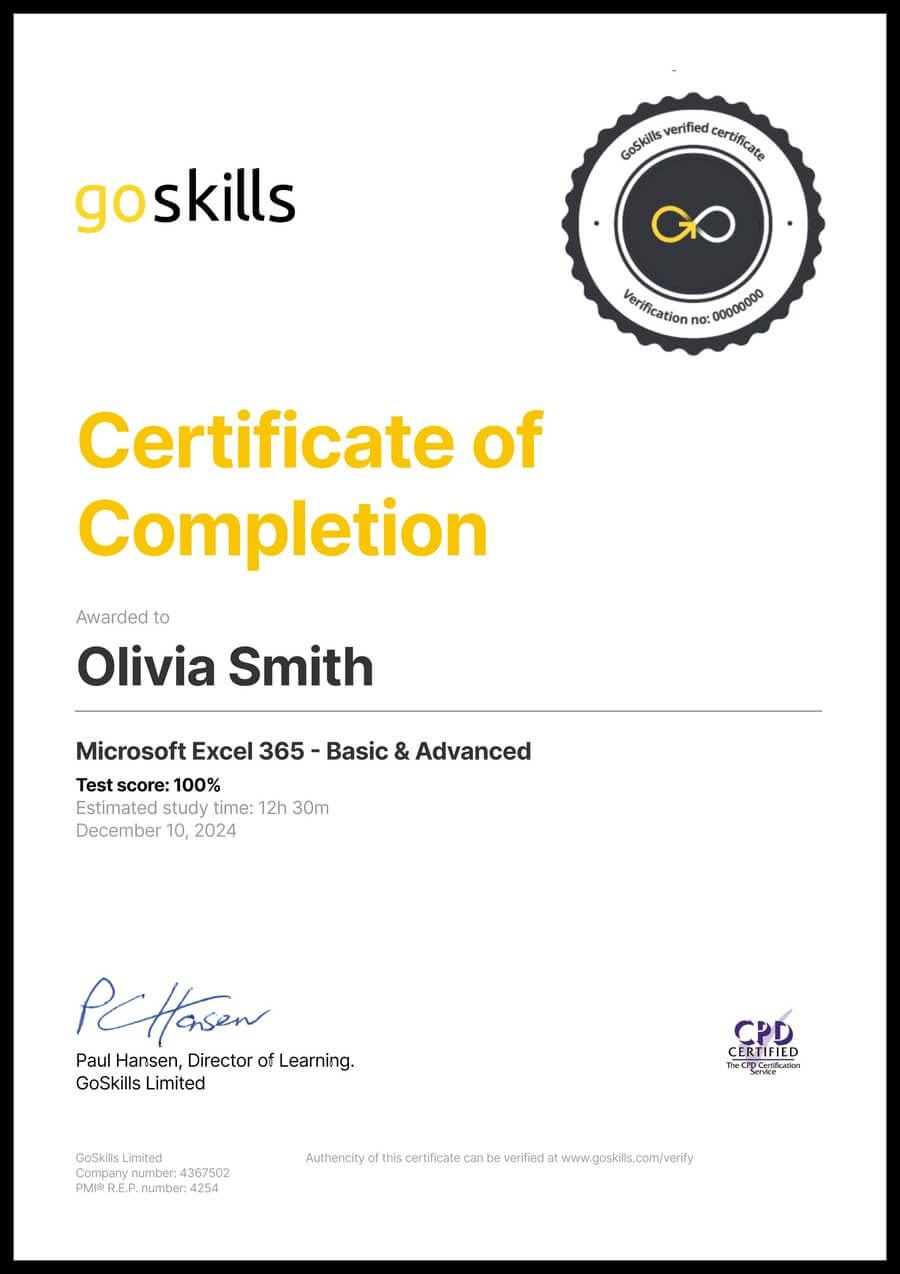
Instructor
John Elder
John founded one of the Internet's earliest advertising networks (bannerclicks.com) and sold it at the height of the first dot com boom. John went on to develop the award-winning Submission-Spider search engine submission software that's been used by over 3 million individuals, businesses, and governments in over 42 countries.
John has over 20 years experience in web development, building professional websites across all platforms. John's passion for learning new technologies lead him to master both front end and back end work, making him a sought after full-stack developer.
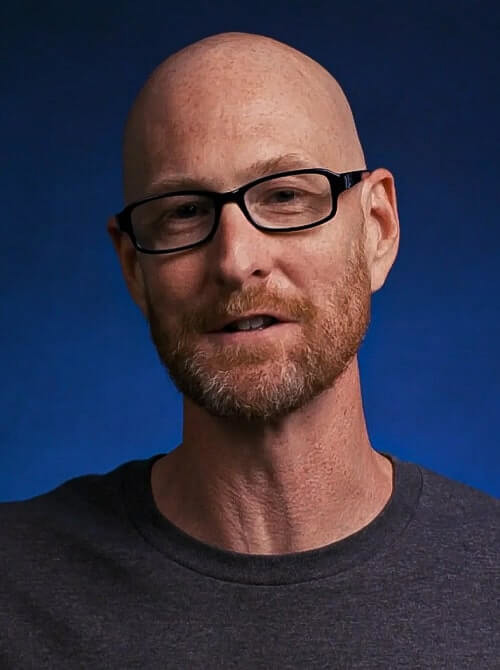
John Elder
Web Developer and Author
Accreditations
Link to awardsHow GoSkills helped Chris
I got the promotion largely because of the skills I could develop, thanks to the GoSkills courses I took. I set aside at least 30 minutes daily to invest in myself and my professional growth. Seeing how much this has helped me become a more efficient employee is a big motivation.
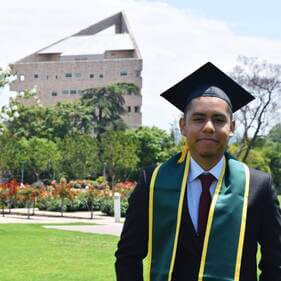